First Class Functions
Please Log In for full access to the web site.
Note that this link will take you to an external site (https://shimmer.mit.edu) to authenticate, and then you will be redirected back to this page.
Consider the below program, separated into pieces. Indicate the value of the prompted expressions. If evaluating the expression would cause an error, write error in the box. If an expression is a function, write function in the box.
An environment diagram may be particularly helpful here.
Note: the single underscore is a valid variable name, often used to indicate a variable that is not explicitly used anywhere.
Relevant Readings about functions that return functions.
def get_multi_applicator(func, n):
"""
Arguments
func: a function of one argument
n: the integer number of times to apply func
Returns a function which applies func to its single
argument n times, repeatedly
"""
def multi_applicator(arg):
for _ in range(n):
func(arg)
return multi_applicator
def double_dict_values(d):
for key in d:
d[key] = 2 * d[key]
x = get_multi_applicator(double_dict_values, 2)
What's the value of x
?
d = {'a': 1, 'b': 2}
y = x(d)
What's the value of y
?
What's the value of d
?
def foo(arg):
arg.append(4)
z = get_multi_applicator(foo, 3)
L = [1, 2, 3]
w = z(L)
What's the value of w
?
What's the value of L
?
x = []
def outer():
x = []
def inner(y):
x.append(y)
return x
return inner
f = outer()
z = f(4)
t = f(3)
w = outer()(2)
print(x)
What's the value of z
?
What's the value of w
?
What's the value of x
that gets printed in line 13?
This result might seem surprising, but the environment diagram below that shows the program up to line 11 should hopefully make things a bit clearer:
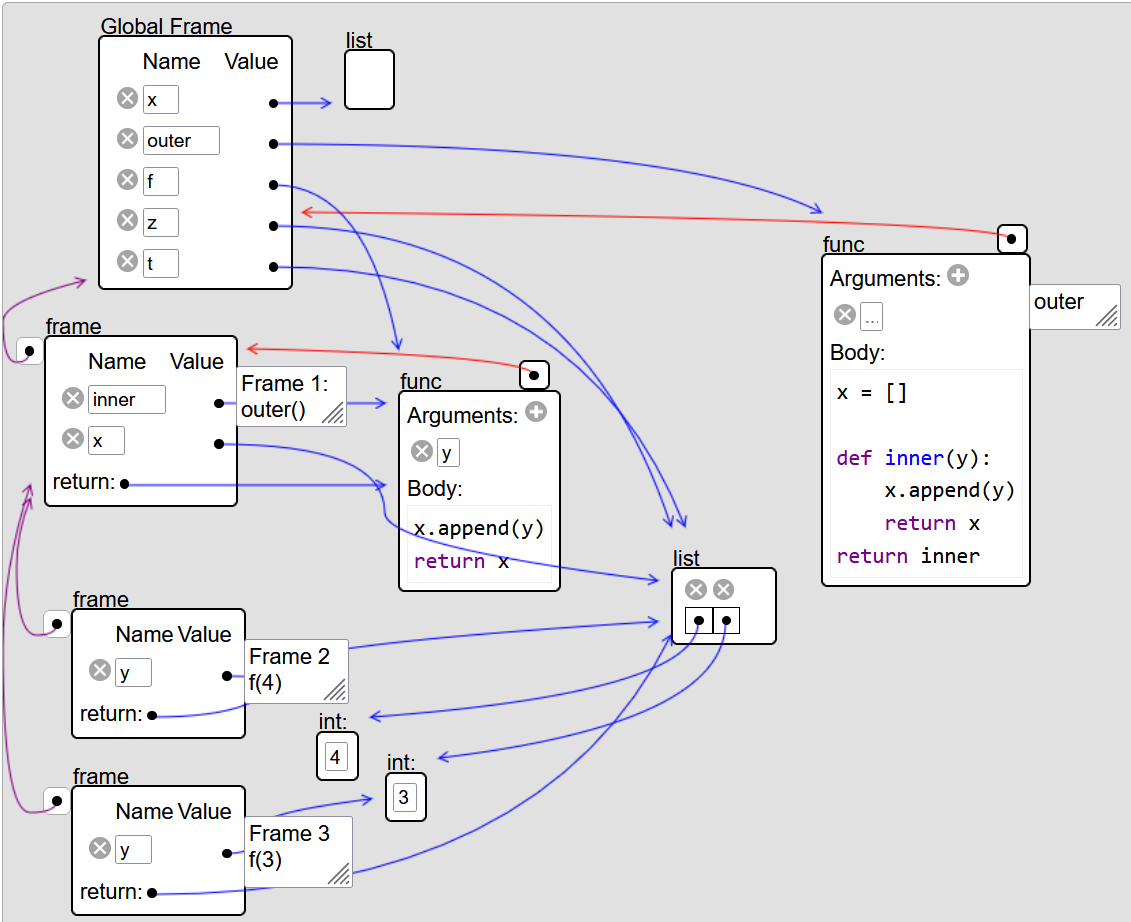
Next Exercise: Dictionary Map